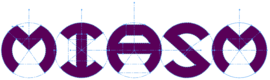 |
miasm
Reverse engineering framework
|
Go to the documentation of this file. 1 #ifndef __COMPAT_PY23_H__
2 #define __COMPAT_PY23_H__
7 #if PY_MAJOR_VERSION >= 3
8 #define PyGetInt_uint_t(size_type, item, value) \
9 if (PyLong_Check(item)) { \
11 PyObject* py_long = item; \
12 PyObject* py_long_new; \
17 if (Py_SIZE(py_long) < 0) { \
19 py_long_new = PyObject_CallMethod(py_long, "__neg__", NULL); \
21 py_long = py_long_new; \
24 bn = PyLong_to_bn(py_long); \
26 bn_t mask_bn = bignum_lshift(bignum_from_int(1), sizeof(size_type)*8); \
27 if (bignum_is_inf_equal_unsigned(mask_bn, bn)) { \
28 RAISE(PyExc_TypeError, "Arg too big for " #size_type ""); \
31 bn = bignum_sub(mask_bn, bn); \
33 tmp = bignum_to_uint64(bn); \
34 value = (size_type) tmp; \
37 RAISE(PyExc_TypeError, "Arg must be int"); \
41 #define PyGetInt_uint_t_retneg(size_type, item, value) \
42 if (PyLong_Check(item)) { \
44 PyObject* py_long = item; \
45 PyObject* py_long_new; \
50 if (Py_SIZE(py_long) < 0) { \
52 py_long_new = PyObject_CallMethod(py_long, "__neg__", NULL); \
54 py_long = py_long_new; \
57 bn = PyLong_to_bn(py_long); \
59 bn_t mask_bn = bignum_lshift(bignum_from_int(1), sizeof(size_type)*8); \
60 if (bignum_is_inf_equal_unsigned(mask_bn, bn)) { \
61 PyErr_SetString(PyExc_TypeError, "Arg too big for " #size_type ""); \
65 bn = bignum_sub(mask_bn, bn); \
67 tmp = bignum_to_uint64(bn); \
68 value = (size_type) tmp; \
71 PyErr_SetString(PyExc_TypeError, "Arg must be int"); \
75 #define PyGetStr(dest, name) \
76 if (!PyUnicode_Check((name))) \
77 RAISE(PyExc_TypeError,"Page name must be bytes"); \
78 (dest) = PyUnicode_AsUTF8((name))
83 #define PyGetInt_uint_t(size_type, item, value) \
84 if (PyInt_Check(item)) { \
86 tmp = PyInt_AsLong(item); \
88 if (Py_SIZE(item) < 0) { \
89 if (-tmp > ((size_type) -1)) { \
90 RAISE(PyExc_TypeError, "Arg too big for " #size_type ""); \
93 else if (tmp > (size_type) -1) { \
94 RAISE(PyExc_TypeError, "Arg too big for " #size_type ""); \
96 value = (size_type) tmp; \
98 else if (PyLong_Check(item)){ \
100 PyObject* py_long = item; \
101 PyObject* py_long_new; \
106 if (Py_SIZE(py_long) < 0) { \
108 py_long_new = PyObject_CallMethod(py_long, "__neg__", NULL); \
109 Py_DECREF(py_long); \
110 py_long = py_long_new; \
113 bn = PyLong_to_bn(py_long); \
115 bn_t mask_bn = bignum_lshift(bignum_from_int(1), sizeof(size_type)*8); \
116 if (bignum_is_inf_equal_unsigned(mask_bn, bn)) { \
117 RAISE(PyExc_TypeError, "Arg too big for " #size_type ""); \
120 bn = bignum_sub(mask_bn, bn); \
122 tmp = bignum_to_uint64(bn); \
123 value = (size_type) tmp; \
126 RAISE(PyExc_TypeError, "Arg must be int"); \
130 #define PyGetInt_uint_t_retneg(size_type, item, value) \
131 if (PyInt_Check(item)) { \
133 tmp = PyInt_AsLong(item); \
135 if (Py_SIZE(item) < 0) { \
136 if (-tmp > ((size_type) -1)) { \
137 PyErr_SetString(PyExc_TypeError, "Arg too big for " #size_type ""); \
141 else if (tmp > (size_type) -1) { \
142 PyErr_SetString(PyExc_TypeError, "Arg too big for " #size_type ""); \
145 value = (size_type) tmp; \
147 else if (PyLong_Check(item)){ \
149 PyObject* py_long = item; \
150 PyObject* py_long_new; \
155 if (Py_SIZE(py_long) < 0) { \
157 py_long_new = PyObject_CallMethod(py_long, "__neg__", NULL); \
158 Py_DECREF(py_long); \
159 py_long = py_long_new; \
162 bn = PyLong_to_bn(py_long); \
164 bn_t mask_bn = bignum_lshift(bignum_from_int(1), sizeof(size_type)*8); \
165 if (bignum_is_inf_equal_unsigned(mask_bn, bn)) { \
166 PyErr_SetString(PyExc_TypeError, "Arg too big for " #size_type ""); \
170 bn = bignum_sub(mask_bn, bn); \
172 tmp = bignum_to_uint64(bn); \
173 value = (size_type) tmp; \
176 PyErr_SetString(PyExc_TypeError, "Arg must be int"); \
181 #define PyGetStr(dest, name) \
182 if (!PyString_Check((name))) \
183 RAISE(PyExc_TypeError,"Page name must be bytes"); \
184 (dest) = PyString_AsString((name))
190 #define PyGetInt_size_t(item, value) PyGetInt_uint_t(size_t, item, value)
192 #define PyGetInt_uint8_t(item, value) PyGetInt_uint_t(uint8_t, item, value)
193 #define PyGetInt_uint16_t(item, value) PyGetInt_uint_t(uint16_t, item, value)
194 #define PyGetInt_uint32_t(item, value) PyGetInt_uint_t(uint32_t, item, value)
195 #define PyGetInt_uint64_t(item, value) PyGetInt_uint_t(uint64_t, item, value)
197 #define PyGetInt_uint8_t_retneg(item, value) PyGetInt_uint_t_retneg(uint8_t, item, value)
198 #define PyGetInt_uint16_t_retneg(item, value) PyGetInt_uint_t_retneg(uint16_t, item, value)
199 #define PyGetInt_uint32_t_retneg(item, value) PyGetInt_uint_t_retneg(uint32_t, item, value)
200 #define PyGetInt_uint64_t_retneg(item, value) PyGetInt_uint_t_retneg(uint64_t, item, value)
204 #if PY_MAJOR_VERSION >= 3
206 #define MOD_INIT(name) PyMODINIT_FUNC PyInit_##name(void)
208 #define MOD_DEF(ob, name, doc, methods) \
209 static struct PyModuleDef moduledef = { \
210 PyModuleDef_HEAD_INIT, name, doc, -1, methods, }; \
211 ob = PyModule_Create(&moduledef);
212 #define RET_MODULE return module
216 #define MOD_INIT(name) PyMODINIT_FUNC init##name(void)
218 #define MOD_DEF(ob, name, doc, methods) \
219 ob = Py_InitModule3(name, methods, doc);
221 #define RET_MODULE return
def traverse_expr_dst(self, expr, dst2index)
Definition: codegen.py:325
def dst_to_c(self, src)
Definition: codegen.py:124
def __init__(self, log_mn=False, log_regs=False)
Definition: codegen.py:33
def id_to_c(self, expr)
Definition: codegen.py:134
def gen_finalize(self, block)
Definition: codegen.py:615
def gen_c_assignments(self, assignblk)
Definition: codegen.py:229
Definition: expression.py:1
translator
Definition: codegen.py:113
string CODE_CPU_EXCEPTION_POST_INSTR
Definition: codegen.py:79
def gen_dst_goto(self, attrib, instr_offsets, dst2index)
Definition: codegen.py:442
def gen_goto_code(self, attrib, instr_offsets, dst)
Definition: codegen.py:409
def gen_post_instr_checks(self, attrib)
Definition: codegen.py:370
def gen_check_cpu_exception(self, address)
Definition: codegen.py:319
Definition: expression.py:1103
log_regs
Definition: codegen.py:38
id_to_c_id
Definition: codegen.py:118
Definition: expression.py:816
Definition: asmblock.py:1
def get_attributes(self, instr, irblocks, log_mn=False, log_regs=False)
Definition: codegen.py:536
Definition: codegen.py:27
def gen_assignblk_dst(self, dst)
Definition: codegen.py:359
string CODE_VM_EXCEPTION_POST_INSTR
Definition: codegen.py:87
string CODE_RETURN_NO_EXCEPTION
Definition: codegen.py:72
def patch_c_id(self, expr)
Definition: codegen.py:130
def add_local_var(self, dst_var, dst_index, expr)
Definition: codegen.py:188
def gen_post_code(self, attrib, pc_value)
Definition: codegen.py:399
PC
Definition: codegen.py:112
def gen_c_code(self, attrib, c_dst, c_assignmnts)
Definition: codegen.py:472
C_PC
Definition: codegen.py:122
def gen_irblock(self, instr_attrib, attributes, instr_offsets, irblock)
Definition: codegen.py:590
string CODE_EXCEPTION_MEM_AT_INSTR
Definition: codegen.py:51
def gen_pre_code(self, instr_attrib)
Definition: codegen.py:384
def __init__(self, ir_arch)
Definition: codegen.py:110
mem_read
Definition: codegen.py:34
def is_expr(expr)
Definition: expression.py:141
Definition: simplifications.py:1
Definition: expression.py:742
set_exception
Definition: codegen.py:36
def gen_bad_block(self)
Definition: codegen.py:563
def init_arch_C(self)
Definition: codegen.py:116
log_mn
Definition: codegen.py:37
def block2assignblks(self, block)
Definition: codegen.py:161
string CODE_BAD_BLOCK
Definition: codegen.py:105
ir_arch
Definition: codegen.py:111
def get_mem_prefetch(self, assignblk)
Definition: codegen.py:206
Definition: codegen.py:42
def gen_check_memory_exception(self, address)
Definition: codegen.py:313
string CODE_EXCEPTION_AT_INSTR
Definition: codegen.py:60
def assignblk_to_irbloc(self, instr, assignblk)
Definition: codegen.py:146
def get_caracteristics(self, assignblk, attrib)
Definition: codegen.py:518
def gen_init(self, block)
Definition: codegen.py:577
def add_label_index(self, dst2index, loc_key)
Definition: codegen.py:138
mem_write
Definition: codegen.py:35
string CODE_INIT
Definition: codegen.py:97
Definition: expression.py:879
instr
Definition: codegen.py:39
def gen_c(self, block, log_mn=False, log_regs=False)
Definition: codegen.py:626
def get_block_post_label(self, block)
Definition: codegen.py:569