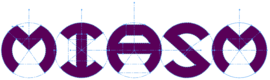 |
miasm
Reverse engineering framework
|
Go to the documentation of this file.
5 #define _MIASM_EXPORT __declspec(dllexport)
43 #define BN_BYTE_SIZE 32
45 #define BN_BIT_SIZE ((BN_BYTE_SIZE) * 8)
49 #define BN_ARRAY_SIZE (BN_BYTE_SIZE / WORD_SIZE)
55 #error Must define WORD_SIZE to be 1, 2, 4
56 #elif (WORD_SIZE == 1)
59 #define DTYPE_SIGNED int8_t
61 #define DTYPE_MSB ((DTYPE_TMP)(0x80))
63 #define DTYPE_TMP uint32_t
65 #define SPRINTF_FORMAT_STR "%.02x"
66 #define SSCANF_FORMAT_STR "%2hhx"
68 #define MAX_VAL ((DTYPE_TMP)0xFF)
69 #elif (WORD_SIZE == 2)
70 #define DTYPE uint16_t
71 #define DTYPE_SIGNED int16_t
72 #define DTYPE_TMP uint32_t
73 #define DTYPE_MSB ((DTYPE_TMP)(0x8000))
74 #define SPRINTF_FORMAT_STR "%.04x"
75 #define SSCANF_FORMAT_STR "%4hx"
76 #define MAX_VAL ((DTYPE_TMP)0xFFFF)
77 #elif (WORD_SIZE == 4)
78 #define DTYPE uint32_t
79 #define DTYPE_SIGNED int32_t
80 #define DTYPE_TMP uint64_t
81 #define DTYPE_MSB ((DTYPE_TMP)(0x80000000))
82 #define SPRINTF_FORMAT_STR "%.08x"
83 #define SSCANF_FORMAT_STR "%8x"
84 #define MAX_VAL ((DTYPE_TMP)0xFFFFFFFF)
87 #error DTYPE must be defined to uint8_t, uint16_t uint32_t or whatever
92 #define require(p, msg) assert(p && #msg)
_MIASM_EXPORT bn_t bignum_init(void)
Definition: bn.c:41
_MIASM_EXPORT bn_t bignum_from_uint64(uint64_t i)
Definition: bn.c:82
bn_t bignum_rshift(bn_t a, int nbits)
Definition: bn.c:416
#define BN_ARRAY_SIZE
Definition: bn.h:49
int bignum_cmp_unsigned(bn_t a, bn_t b)
Definition: bn.c:602
_MIASM_EXPORT bn_t bignum_dec(bn_t n)
Definition: bn.c:218
res
Definition: regs.py:71
int bignum_cmp_signed(bn_t a, bn_t b)
Definition: bn.c:583
int bignum_getbit(bn_t a, int pos)
Definition: bn.c:820
bn_t bignum_add(bn_t a, bn_t b)
Definition: bn.c:263
_MIASM_EXPORT bn_t bignum_add(bn_t a, bn_t b)
Definition: bn.c:263
_MIASM_EXPORT bn_t bignum_xor(bn_t a, bn_t b)
Definition: bn.c:546
#define require(p, msg)
Definition: bn.h:92
_MIASM_EXPORT int bignum_cntleadzeros(bn_t n, int size)
Definition: bn.c:842
bn_t bignum_assign(bn_t src)
Definition: bn.c:684
_MIASM_EXPORT bn_t bignum_mask(bn_t src, int bits)
Definition: bn.c:699
bn_t bignum_smod(bn_t a, bn_t b, int size)
Definition: bn.c:921
_MIASM_EXPORT bn_t bignum_mul(bn_t a, bn_t b)
Definition: bn.c:309
_MIASM_EXPORT int bignum_to_int(bn_t n)
Definition: bn.c:111
bn_t bignum_rol(bn_t a, int size, int nbits)
Definition: bn.c:794
#define SPRINTF_FORMAT_STR
Definition: bn.h:82
_MIASM_EXPORT bn_t bignum_udiv(bn_t a, bn_t b)
Definition: bn.c:341
#define _MIASM_EXPORT
Definition: bn.h:7
_MIASM_EXPORT bn_t bignum_smod(bn_t a, bn_t b, int size)
Definition: bn.c:921
int bignum_is_equal(bn_t a, bn_t b)
Definition: bn.c:609
bn_t bignum_or(bn_t a, bn_t b)
Definition: bn.c:531
bn_t bignum_a_rshift(bn_t a, int size, int nbits)
Definition: bn.c:446
bn_t bignum_mul(bn_t a, bn_t b)
Definition: bn.c:309
int bignum_cnttrailzeros(bn_t n, int size)
Definition: bn.c:867
i
Definition: modint.py:70
int bignum_cntleadzeros(bn_t n, int size)
Definition: bn.c:842
_MIASM_EXPORT bn_t bignum_a_rshift(bn_t a, int size, int nbits)
Definition: bn.c:446
_MIASM_EXPORT bn_t bignum_and(bn_t a, bn_t b)
Definition: bn.c:515
int bignum_is_zero(bn_t n)
Definition: bn.c:668
_MIASM_EXPORT bn_t bignum_assign(bn_t src)
Definition: bn.c:684
d
Definition: basic_op.py:30
int bignum_is_inf_signed(bn_t a, bn_t b)
Definition: bn.c:645
DTYPE array[BN_ARRAY_SIZE]
Definition: bn.h:98
#define MAX_VAL
Definition: bn.h:84
def ret(arg1)
Definition: sem.py:1796
bn_t bignum_inc(bn_t n)
Definition: bn.c:240
_MIASM_EXPORT int bignum_is_zero(bn_t n)
Definition: bn.c:668
bn_t bignum_udiv(bn_t a, bn_t b)
Definition: bn.c:341
c
Definition: basic_op.py:15
#define BN_BIT_SIZE
Definition: bn.h:45
_MIASM_EXPORT bn_t bignum_sub(bn_t a, bn_t b)
Definition: bn.c:283
#define WORD_SIZE
Definition: bn.h:40
bn_t bignum_from_uint64(uint64_t i)
Definition: bn.c:82
bn_t bignum_umod(bn_t a, bn_t b)
Definition: bn.c:493
_MIASM_EXPORT bn_t bignum_umod(bn_t a, bn_t b)
Definition: bn.c:493
_MIASM_EXPORT bn_t bignum_lshift(bn_t a, int nbits)
Definition: bn.c:387
def j(arg1)
Definition: sem.py:156
bn_t bignum_dec(bn_t n)
Definition: bn.c:218
_MIASM_EXPORT bn_t bignum_rol(bn_t a, int size, int nbits)
Definition: bn.c:794
_MIASM_EXPORT int bignum_cnttrailzeros(bn_t n, int size)
Definition: bn.c:867
@ SMALLER
Definition: bn.h:104
_MIASM_EXPORT int bignum_is_inf_equal_signed(bn_t a, bn_t b)
Definition: bn.c:657
_MIASM_EXPORT bn_t bignum_inc(bn_t n)
Definition: bn.c:240
_MIASM_EXPORT bn_t bignum_sdiv(bn_t a, bn_t b, int size)
Definition: bn.c:885
@ EQUAL
Definition: bn.h:104
tuple mask
Definition: modularintervals.py:32
_MIASM_EXPORT int bignum_cmp(bn_t a, bn_t b)
Definition: bn.c:561
#define DTYPE
Definition: bn.h:78
bn_t bignum_ror(bn_t a, int size, int nbits)
Definition: bn.c:807
_MIASM_EXPORT bn_t bignum_from_string(char *str, int nbytes)
Definition: bn.c:168
bn_t bignum_from_int(DTYPE_TMP i)
Definition: bn.c:54
_MIASM_EXPORT bn_t bignum_or(bn_t a, bn_t b)
Definition: bn.c:531
_MIASM_EXPORT uint64_t bignum_to_uint64(bn_t n)
Definition: bn.c:134
bn_t bignum_from_string(char *str, int nbytes)
Definition: bn.c:168
bn_t bignum_sub(bn_t a, bn_t b)
Definition: bn.c:283
#define DTYPE_SIGNED
Definition: bn.h:79
_MIASM_EXPORT int bignum_is_inf_signed(bn_t a, bn_t b)
Definition: bn.c:645
_MIASM_EXPORT bn_t bignum_rshift(bn_t a, int nbits)
Definition: bn.c:416
bn_t bignum_not(bn_t a)
Definition: bn.c:479
int bignum_is_inf_equal_signed(bn_t a, bn_t b)
Definition: bn.c:657
bn_t bignum_lshift(bn_t a, int nbits)
Definition: bn.c:387
_MIASM_EXPORT int bignum_is_equal(bn_t a, bn_t b)
Definition: bn.c:609
_MIASM_EXPORT bn_t bignum_from_int(DTYPE_TMP i)
Definition: bn.c:54
_MIASM_EXPORT int bignum_getbit(bn_t a, int pos)
Definition: bn.c:820
int bignum_to_int(bn_t n)
Definition: bn.c:111
uint64_t bignum_to_uint64(bn_t n)
Definition: bn.c:134
bn_t bignum_mask(bn_t src, int bits)
Definition: bn.c:699
_MIASM_EXPORT bn_t bignum_not(bn_t a)
Definition: bn.c:479
@ LARGER
Definition: bn.h:104
_MIASM_EXPORT int bignum_is_inf_equal_unsigned(bn_t a, bn_t b)
Definition: bn.c:633
int bignum_is_inf_unsigned(bn_t a, bn_t b)
Definition: bn.c:621
int bignum_is_inf_equal_unsigned(bn_t a, bn_t b)
Definition: bn.c:633
bn_t bignum_init(void)
Definition: bn.c:41
void bignum_to_string(bn_t n, char *str, int nbytes)
Definition: bn.c:196
bn_t bignum_xor(bn_t a, bn_t b)
Definition: bn.c:546
bn_t bignum_sdiv(bn_t a, bn_t b, int size)
Definition: bn.c:885
_MIASM_EXPORT void bignum_to_string(bn_t n, char *str, int maxsize)
Definition: bn.c:196
a
Definition: basic_op.py:9
#define SSCANF_FORMAT_STR
Definition: bn.h:83
size
Definition: shellcode.py:32
b
Definition: basic_op.py:10
bn_t bignum_and(bn_t a, bn_t b)
Definition: bn.c:515
_MIASM_EXPORT int bignum_is_inf_unsigned(bn_t a, bn_t b)
Definition: bn.c:621
int bignum_cmp(bn_t a, bn_t b)
Definition: bn.c:561
#define DTYPE_TMP
Definition: bn.h:80
_MIASM_EXPORT bn_t bignum_ror(bn_t a, int size, int nbits)
Definition: bn.c:807